
Prompt with current result below:
Can you draw a cityscape at night with a moon, stars and lights on in the building windows, with the Gorilla from the video game Rampage climbing the far right building in javascript?
Result had a couple problems:
1) Was missing end tags:
</script>
</body>
</html>
2) The Gorilla was basically a little peg man standing on top of the building:
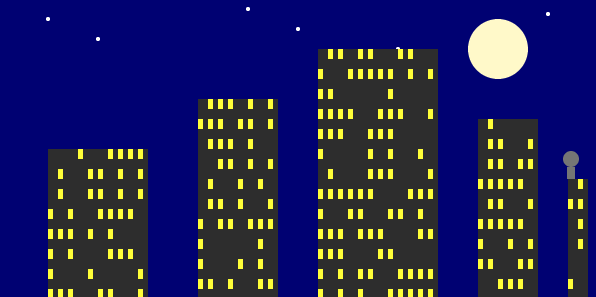
Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Cityscape at Night with Rampage Gorilla</title>
<style>
canvas {
display: block;
margin: 0 auto;
background-color: #f0f0f0;
}
</style>
</head>
<body>
<canvas id="rampageCityCanvas" width="600" height="300"></canvas>
<script>
const canvas = document.getElementById('rampageCityCanvas');
const ctx = canvas.getContext('2d');
// Night sky background
ctx.fillStyle = '#000080';
ctx.fillRect(0, 0, canvas.width, canvas.height);
// Draw the moon
ctx.fillStyle = '#FFFACD';
ctx.beginPath();
ctx.arc(500, 50, 30, 0, Math.PI * 2);
ctx.fill();
// Draw stars
ctx.fillStyle = '#FFFFFF';
const stars = [
{ x: 50, y: 20 }, { x: 100, y: 40 }, { x: 250, y: 10 },
{ x: 300, y: 30 }, { x: 400, y: 50 }, { x: 550, y: 15 },
];
for (const star of stars) {
ctx.beginPath();
ctx.arc(star.x, star.y, 2, 0, Math.PI * 2);
ctx.fill();
}
// Draw buildings
const buildings = [
{ x: 50, y: 150, width: 100, height: 150 },
{ x: 200, y: 100, width: 80, height: 200 },
{ x: 320, y: 50, width: 120, height: 250 },
{ x: 480, y: 120, width: 60, height: 180 },
{ x: 570, y: 180, width: 20, height: 120 },
];
ctx.fillStyle = '#333';
for (const building of buildings) {
ctx.fillRect(building.x, building.y, building.width, building.height);
}
// Draw windows with lights
ctx.fillStyle = '#FFFF00';
for (const building of buildings) {
for (let i = 0; i < building.width; i += 10) {
for (let j = 0; j < building.height; j += 20) {
if (Math.random() < 0.5) {
ctx.fillRect(building.x + i, building.y + j, 5, 10);
}
}
}
}
// Draw the Rampage Gorilla
const gorillaX = 573;
const gorillaY = 160;
ctx.fillStyle = '#808080';
ctx.beginPath();
ctx.arc(gorillaX, gorillaY, 8, 0, Math.PI * 2); // head
ctx.fill();
ctx.fillRect(gorillaX - 4, gorillaY + 8, 8, 12); // body
If ChatGPT / GPT-4 can successfully accomplish the above in a one-shot answer by the end of 2023, this resolves as YES.
Other, "Breaking LLM's Markets"
🏅 Top traders
# | Name | Total profit |
---|---|---|
1 | Ṁ118 | |
2 | Ṁ8 | |
3 | Ṁ6 | |
4 | Ṁ1 | |
5 | Ṁ0 |
Just did another test myself using Data Analysis powered by GPT-4 and this is what I got.
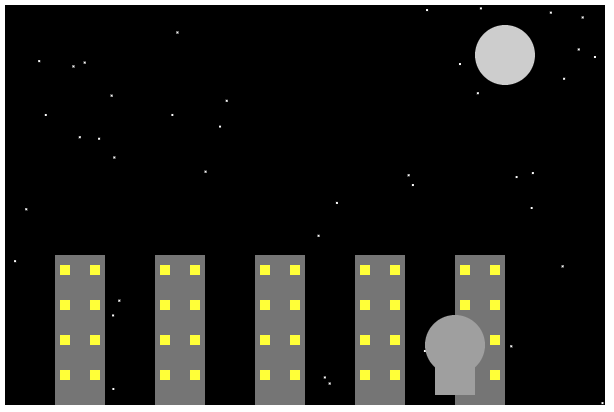
My basis for this still qualifying as GTP-4 is the following under, "about" when you go to select the version of GPT that you are using under, "Explore."
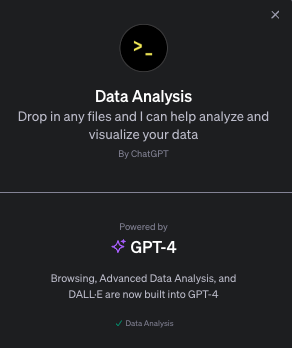
That being said, there is a whole world of different GPT's and the capability to use custom GPT's, so I would say, if someone can make this work via a custom GPT, yet it is still powered by GPT-4, that would qualify.
That being said, the person must be transparent in the comments about how they did it. For example, if you uploaded custom code showing how to render a gorilla as a part of the custom GPT, you have to show that and show how you did it with screenshots. Just merely saying, "here you go," and linking to a chat is not acceptable anymore (unless it's a standard GPT offering) since OpenAI has significantly changed how it operates.
That being said, there may be an existing GPT powered by GPT-4 that I'm not aware of and it would be perfectly acceptable to link to that chat, showing that it works.
The missing end tags thing sounds like it hit the max length. You might want to allow saying "continue" or similar for it to finish the script.
Also: What constitutes a good enough gorilla?
@ShadowyZephyr Since we're getting closer to the end of the year, here is what I will say to try to define this better. Please let me know your thoughts.
Here was the original Rampage Gorilla from 1986.
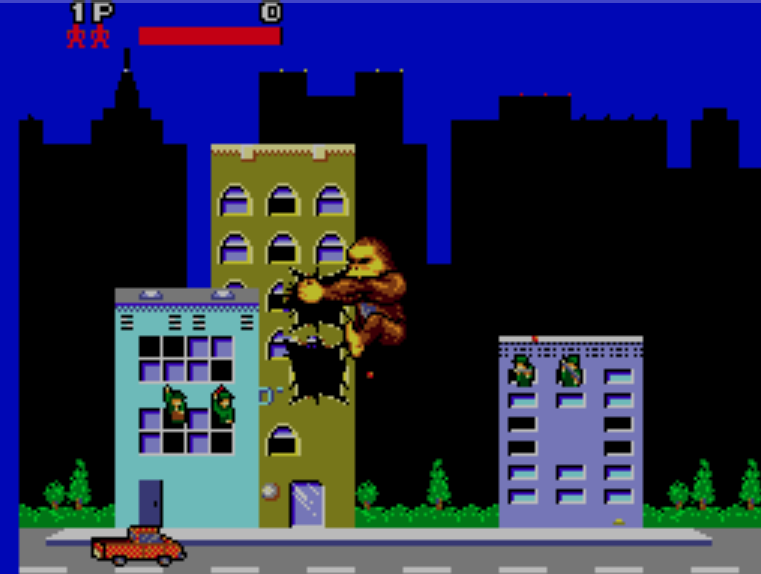
I would say, since we're interested in having this actually coded in Javascript, and we're really just looking for, "significant improvement over the year," as a way to keep up with the times, in line with a lot of the other markets I create, we should be expecting something similar to the first image, but with actual arms and legs at least, hanging on a building.
I don't expect additional colors and layers. I don't expect a street and cars to be rendered. I think what we saw earlier this year might be considered "1970's graphics," and it wouldn't be reasonable to suddenly expect 1986 graphics, but maybe jumping from mid 70's to late 70's or early 80s would be acceptable.
So here's an example of a 1976 game, Sea Wolf:
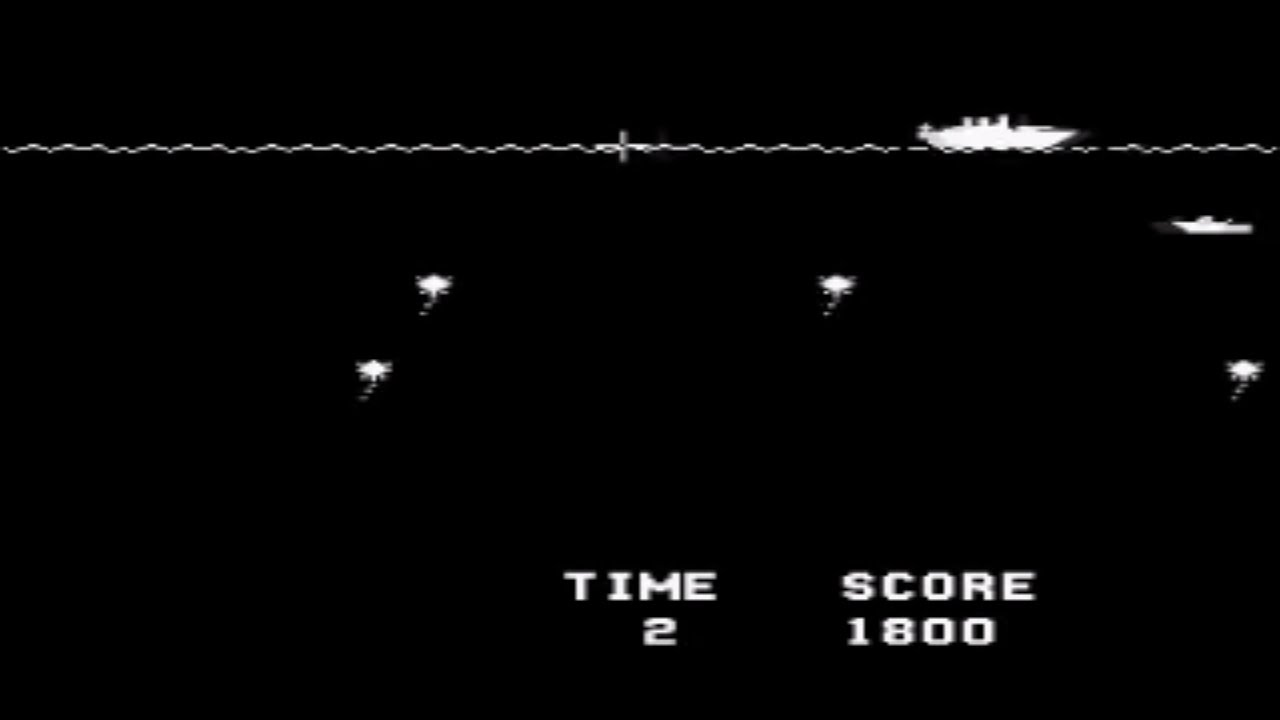
Here was our original image from earlier this year:
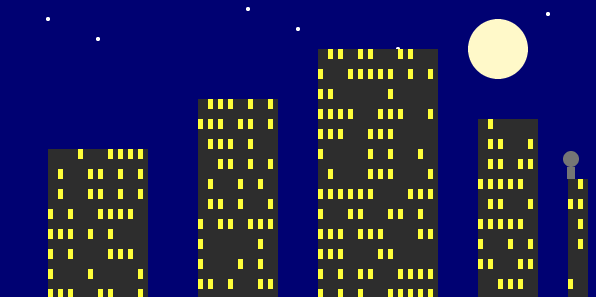
And here's a game from 1980, Rally-X
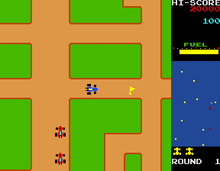
And another one from 1980 Missile Command:
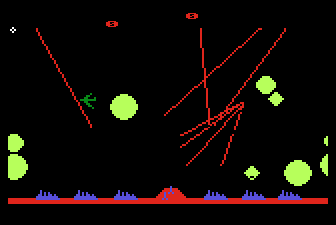
So, I wouldn't expect a massively groundbreaking improvement, like equivalent to 1986 Rampage, but maybe a bit more detailed, some arms and legs showing a figure rather than just a super simple sprite, maybe more colors, like 5 or 6 standout colors rather than 2 or 3. If it shows a hole in one of the buildings that would be a huge positive.
Unfortunately it has to be a bit subjective but I hope this narrows it down a bit. Feel free to ask more questions.