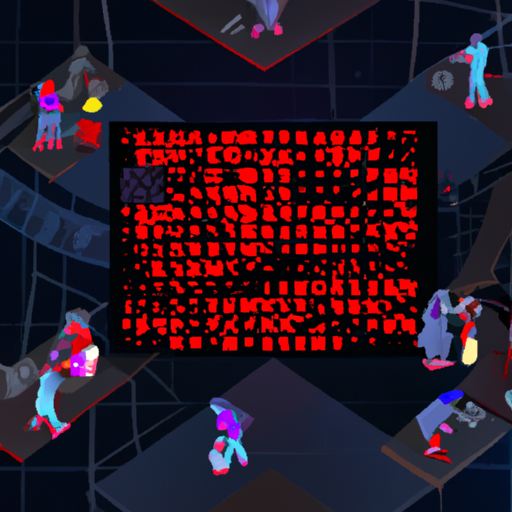
Here's a Python script for determining the average price of "The Market"
import numpy as np
from manifoldpy import api
MARKET_ID = "NRLwgQYqCLk5zTasmxOn"
market = api.get_market(MARKET_ID)
t0 = market.createdTime
tclose = market.closeTime
bets = api.get_all_bets(marketId=MARKET_ID)[::-1]
t = np.array([t0] + [bet.createdTime for bet in bets] + [tclose])
p = 100 * np.array([0.5] + [bet.probAfter for bet in bets])
# rounding:
within_two_of_edges = (p < 2) | (p > 98)
p[within_two_of_edges] = np.round(p[within_two_of_edges], 1)
p[~within_two_of_edges] = np.round(p[~within_two_of_edges], 0)
avg = (p * np.diff(t)).sum() / (t[-1] - t[0])
print(f"Market average is: {avg:.3f}")
It prints:
Fetched 1000 bets.
Fetched 2000 bets.
Fetched 3000 bets.
Fetched 4000 bets.
Fetched 5000 bets.
Fetched 6000 bets.
Fetched 7000 bets.
Fetched 8000 bets.
Fetched 9000 bets.
Fetched 10000 bets.
Fetched 11000 bets.
Fetched 12000 bets.
Fetched 12458 bets.
Market average is: 36.553
As discussed in the comments to the prime number market, this script is giving results inconsistent with other users' calculations.
Will someone explain to me, to my satisfaction, in the comments, precisely why this code is wrong and disagrees with other calculations of the average probability?
If the code turns out to be correct, the market will resolve NO.
Finding a minor bug that changes the result slightly will not be sufficient to resolve the market YES - a YES resolution requires a full explanation of the reason behind disagreement with whatever number is used to resolve The Market.
🏅 Top traders
# | Name | Total profit |
---|---|---|
1 | Ṁ94 | |
2 | Ṁ51 |